Terraform is an infrastructure as code tool that enables you to safely and predictably provision and manage infrastructure in any cloud.
Terraform Overview
Terraform allows you to
- automate and manage your infrastructure
- your platform
- and services that run on that platform
Terraform architecture
Terraform architecture mainly consists of the following components:
- Terraform Core
- Providers
- State file
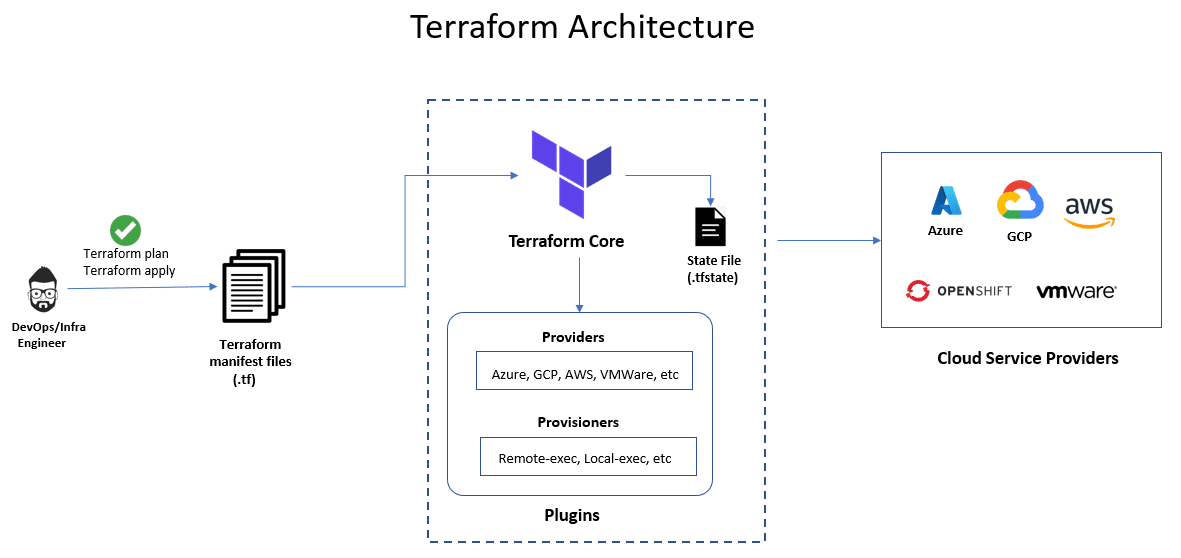
Terraform Structure
Terraform Block
A Terraform block specifies the required providers that terraform needs in order to execute the script. This block also contains the source block that specifies from where terraform should download the provider and also the required version.
1 | # https://developer.hashicorp.com/terraform/language/providers/requirements |
Provider Block
A provider block specifies the cloud provider and the API credentials required to connect to the provider’s services. It includes the provider name, version, access key, and secret key.
1 | # https://registry.terraform.io/providers/hashicorp/aws/latest/docs |
Resource Block
A resource block represents a particular resource in the cloud provider’s services. It includes the resource type, name, and configuration details. This is the main block that specifies the type of resource we are trying to deploy.
1 | # https://developer.hashicorp.com/terraform/language/resources/syntax |
Data Block
A data block is used to fetch data from the provider’s services, which can be used in resource blocks. It includes the data type and configuration details. This is used in scenarios where the resource is already deployed, and you would like to fetch the details of that resource.
1 | # https://developer.hashicorp.com/terraform/language/data-sources |
Variables Block
A variable block is used to define input variables that are used in the Terraform configuration. It includes the variable name, type, and default value.
1 | # https://developer.hashicorp.com/terraform/tutorials/aws-get-started/aws-variables |
Output Block
An output block is used to define output values that are generated by the Terraform configuration. It includes the output name and value.
1 | # https://developer.hashicorp.com/terraform/language/values/outputs |
Modules Block
Modules are containers for multiple resources that are used together. A module consists of .tf
and/or .tf.json
files stored in a directory. It is the primary way to package and reuse resources in Terraform.
Every Terraform configuration has at least one model (root module) which contains resources defined in the .tf files. Test configuration we created in the third part of these series is a module.
Modules are a great way to compartmentalize reusable collections of resources in multiple configurations.
1 | . |
Locals Block
Often called local variables block, this block is used to keep frequently referenced values or expressions to keep the code clean and tidy.
Locals block can hold many variables inside. Expressions in local values are not limited to literal constants. They can also reference other values in the module to transform or combine them. These variables can be accessed using local.var_name
notation, note that it is called local.
when used to access values inside.
1 | # https://developer.hashicorp.com/terraform/language/values/locals |
Using Local Values
1 | resource "aws_instance" "example" { |
Backend Block
A backend defines where Terraform stores its state data files.
1 | terraform { |
Example Referencing
1 | data "terraform_remote_state" "foo" { |
Provisioners Block
Provisioners allows us to specify actions to be performed on local or remote machines to prepare resources for service. There are two types of Terraform provisioners:
local-exec
invokes local executable after a resource is created. It runs the process on the machine running Terraform, meaning the machine where you run terraform apply. This is most likely your own computer.remote-exec
invokes remote executable, something like an EC2 instance on AWS.
This is an example of a provisioner for an EC2 instance. This example contains both local-exec
and a remote-exec
:
1 | resource "aws_instance" "web_server" { |
Providers in Terraform
- Expose resources for specific infrastructure platforms (e.g AWS, Azure, Google Cloud, etc.)
- Responsible for understanding API of that platform
- Just code that knows how to talk to specific technology or platform
1 | # AWS Provider |